PurpleJS is a Javascript application framework running on the Java Virtual Machine. In this post I will tell you what PurpleJS is, why it was created and how to get started.
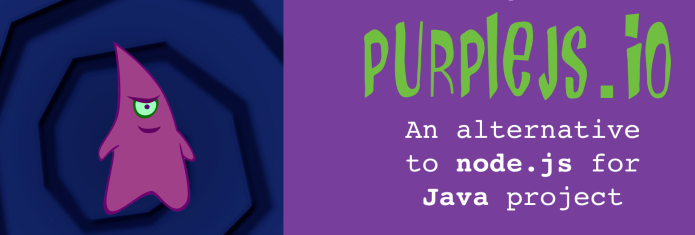
PurpleJS - the alternative to Node.js for Java projects
Okey, so let's say that it's not really necessary to have an alternative to Node.js, but we all do like to have alternatives right? Many many many years ago, when I was only 2^5+8
. I was thinking that it would be nice to combine the power of Java and my existing investments with the simplicity of JavaScript. JavaScript makes it easy to share code between client and server so it's only natural to run JavaScript on the server too. But I wanted at the same time the power of Java and what the JVM can provide.
With Enonic XP, the combination of Java and JavaScript is used to speed up the development process and allow frontend developers to implement backend services. I saw that this was a very popular approach to develop software more effectively, and wanted everyone to be able to use the technology for free and in existing investments. PurpleJS was born.
What is PurpleJS?
PurpleJS is an alternative to Node.js for Java projects. PurpleJS makes it easy to build performant and lightweight Javascript server applications without the complexity of Node.js asynchronous programming model.
PurpleJS gives Javascript developers access to the vast Java community and the robustness and performance of the JVM. Java developers benefit from the simplicity and flexibility of Javascript in their projects.
Use PurpleJS when you want to:
- code Javascript, but your application needs to run on the JVM
- build fast and lightweight multithreaded applications with Javascript
- create server applications with Javascript but access and use Java libraries
- deliver isomorphic applications - running the same code on both client (i.e. browser) and server
- build Javascript server applications that run on any infrastructure
- code fast, no need to compile or re-deploy - especially useful for front-end projects
- extend existing Java applications with Javascript
- participate in the Javascript movement while leveraging your existing projects in Java
PurpleJS is a simple and capable framework for creating performant web applications without leaving Javascript. It is created in Java to give the flexibility and performance Java provides.
Getting started
I'll show you how to get started building your first web-application in PurpleJS.
You need to install two things: Java SDK and Gradle.
Java is needed since PurpleJS is built on Java - that does not mean that you will ever need to program in Java, it's only the runtime. Gradle is a build system that is used for building applications in PurpleJS.
There are multiple ways to create a PurpleJS application, but the fastest is to use PurpleJS Boot. This is a ready-to-go server that's running Jetty and you do not need to worry about anything except your own application logic.
Setting up the project
To set up the project create a directory to hold the project files - like myapp
. Go into the directory and create a file called build.gradle
. This file should contain the following:
To test if this works, start the server by issuing the following command:
If everything works, you will see something like this:
Congratulations, the project is now set up and we can start coding our application. Let's leave the application running. The system will pick up any changes so we do not need to restart the server while coding.
Main application file
If we open our browser and go to http://localhost:8080 we will see an error saying that /app/main.js
is not found. This is our main entry-point for the application and it is required.
So, let's create the file src/main/resources/app/main.js
and add the following:
When we now refresh our browser again (http://localhost:8080), then a Hello World
message appears. Simple, but this illustrates how simple it is to create a controller. You export the HTTP method that should be handled and returns the response. This response has properties like body
, contentType
, headers
and status
. The status is default set to 200
(OK). If we want to return JSON instead, just set a JSON-array or JSON-object to the body
property.
The above example will output { name: 'Bill', age: 60 }
with status = 200
and contentType = 'application/json'
.
Request parameters
Request can contain various information. If you need to see what’s inside the request, just return the entire object as JSON like this:
Let's modify main.js
to implement a random generator that returns a random number between 1 and max. The max parameter should be read from max
request parameter. First, we define the random number function.
Then we modify our exports.get
function to return the random number based on a request parameter.
If we now access http://localhost:8080 without any parameters, we will get a random number between 1 and 10. Requesting http://localhost:8080?max=100 will give a random number between 1 and 100.
Routing
Sooner or later you will find yourself in need of a router. A router can route requests to different controllers and you can split your logic up into multiple files. Let's change our main.js
file to include a router.
This router can be configured by adding one or more routes. Every route can match both HTTP method
and path
. Let's add a route to get a random number.
To make this work we will need to modify our exports.get method so it delegates to the router that we have just configured.
If we now request http://localhost:8080 we will get a 404
since we no longer have a route on /, but on /random. Accessing http://localhost:8080/random will now give us a random number between 1 and 10.
We can also use path parameters to configure a route. If we want to access a random number between 1 and 100 using the /random/100
we can add a route like this:
If you now access http://localhost:8080/random/100 it will give you a random number between 1 and 100.
What's next?
This post is just scratching the surface of what PurpleJS can do. Want to check out what more PurpleJS can do? Please checkout the following resources:
- Main webpage - http://purplejs.io
- Examples - https://github.com/purplejs/purplejs-examples
- GitHub project - https://github.com/purplejs/purplejs
- GitHub wiki - https://github.com/purplejs/purplejs/wiki